本文共 1232 字,大约阅读时间需要 4 分钟。
系列文章目录
前言
C/S 、B/S
C/S:
Client:客户端
Server:服务端
mysql、qq 都是C/S的应用
B/S
Browser:浏览器(也是一个客户端,只不过是一个通用的客户端)
Server:服务器端
严格来说B/S结构也是一种C/S结果只是说它的客户端不是你写的,而是浏览器
比如网页版本的京东、淘宝都是B/S结构
一、网络的概念和分类
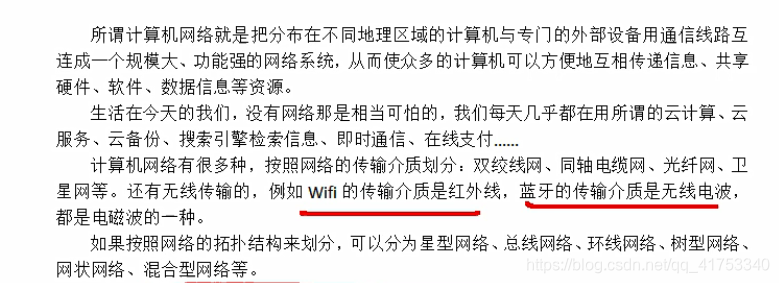
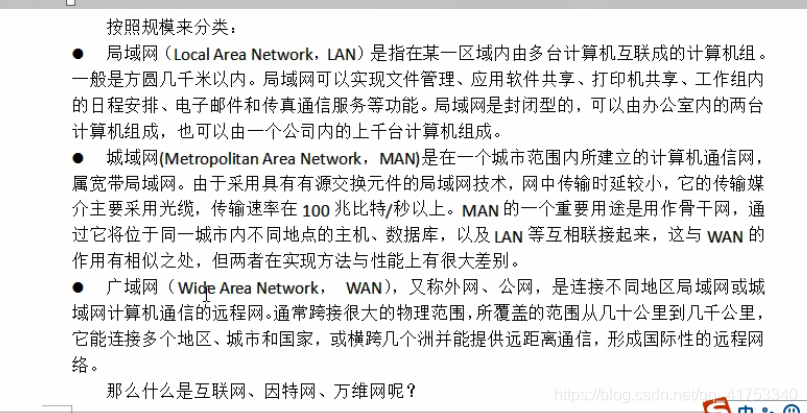
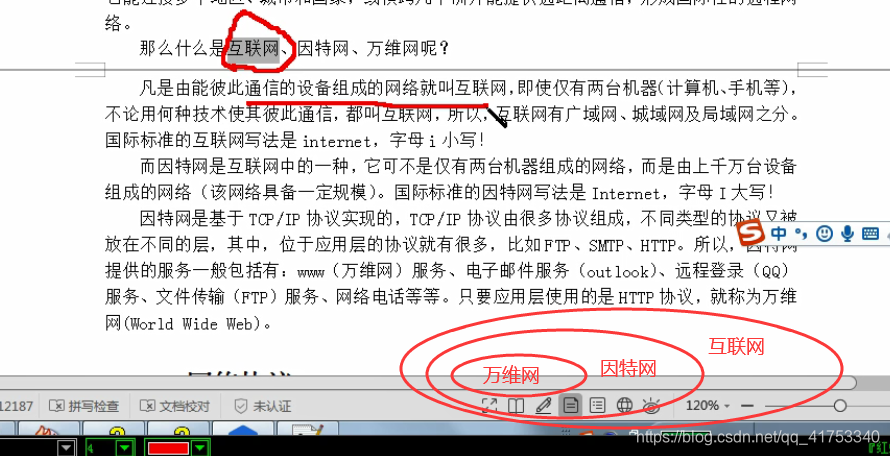
我们所说的外网内网其实都是互联网
我们说的外网,严格来说其实是指因特网
二、网络通讯的三个要输
(1)IP地址
IP地址,定位到一台设备
(2)端口号
定位到一个应用程序(进程)
(3)网络协议
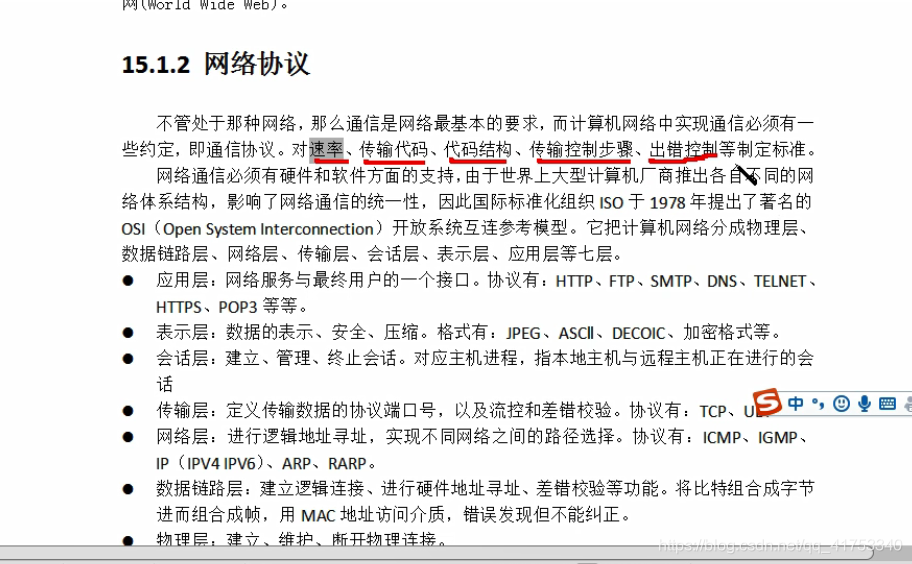
通俗的讲,网络协议就是如何保证数据准确的到达对方那里,并且能够正确的将数据解析出来
由于过于复杂,比如各个厂商生产的网卡也不尽相同,所以国际上最后推出了OSI标准
OSI(Open System Interconnection)开放系统互连参考模型。
它把计算机网络分成物理层、数据链路层、网络层、传输层、会话层、表示层、应用层等七层。
物理层:建立、维护、断开物理连接
数据链路层:建立逻辑连接、进行硬件地址寻址、差错校验等功能。将比特组合成字节进而组合成帧,用MAC地址访问介质,错误发现但不能纠正。
- 网络层:进行逻辑地址寻址,实现不同网络之间的路径选择。协议有:ICMP、IGMP、IP(IPV4 IPV6)、ARP、RARP。
- 传输层:定义传输数据的协议端口号,以及流控和差错校验。协议有:TCP、UDP。
- 会话层:建立、管理、终止会话。对应主机进程,指本地主机与远程主机正在进行的会话
- 表示层:数据的表示、安全、压缩。格式有:JPEG、ASCll、DECOIC、加密格式等
- 应用层:网络服务与最终用户的一个接口。协议有:HTTP、FTP、SMTP、DNS、TELNET、HTTPS、POP3等等。
比如TCP是发错了重传,UDP是发错了就发错了,不重传
实际当中,并不一定是严格的七层,因为七层过于复杂
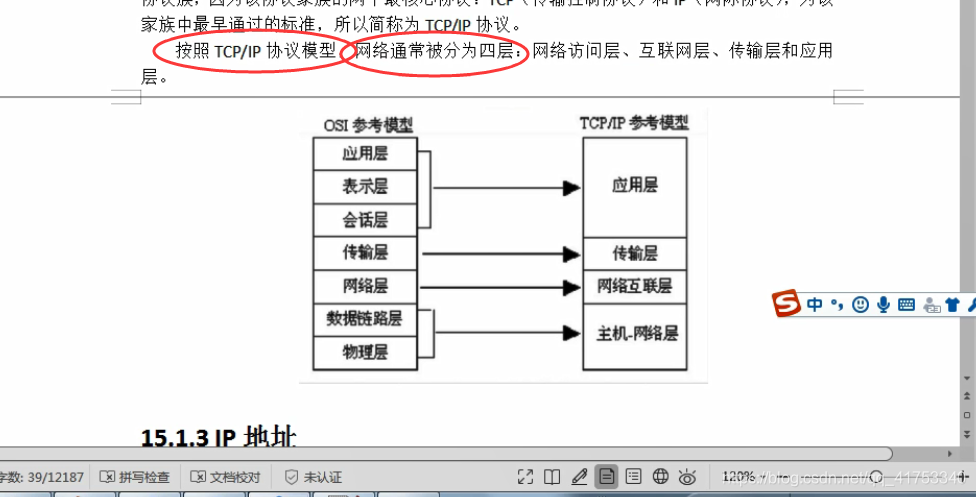
最早确定的,也是最重要的是TCP/IP协议。把这些协议家族称为TCP/IP协议簇。
(1)主机-网路层:硬件层面
(2)网络层:例如IP寻址
(3)传输层:协议有:TCP、UDP
(4)应用层:程序员面对的
TCP:(Transmission Control Protocol,传输控制协议)面向连接的,可靠的,基于字节流的,适用于大数据量的传输的协议。
UDP:(User Datagram Protocol,用户数据报协议)非面向连接,不可靠的,基于用户数据报(报文),只能支持最多64k以内的数据的发送。
三次握手
TCP为什么是可靠的,也是因为这样原因
在发送之前先“对一波暗号”
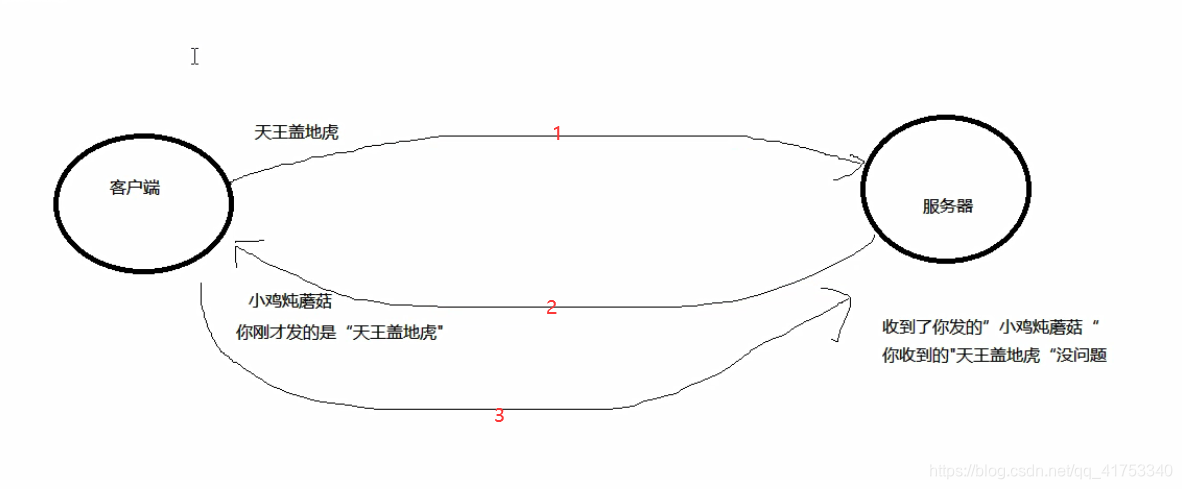
TCP,发消息之前需要三次握手
离开的时候也要四次挥手
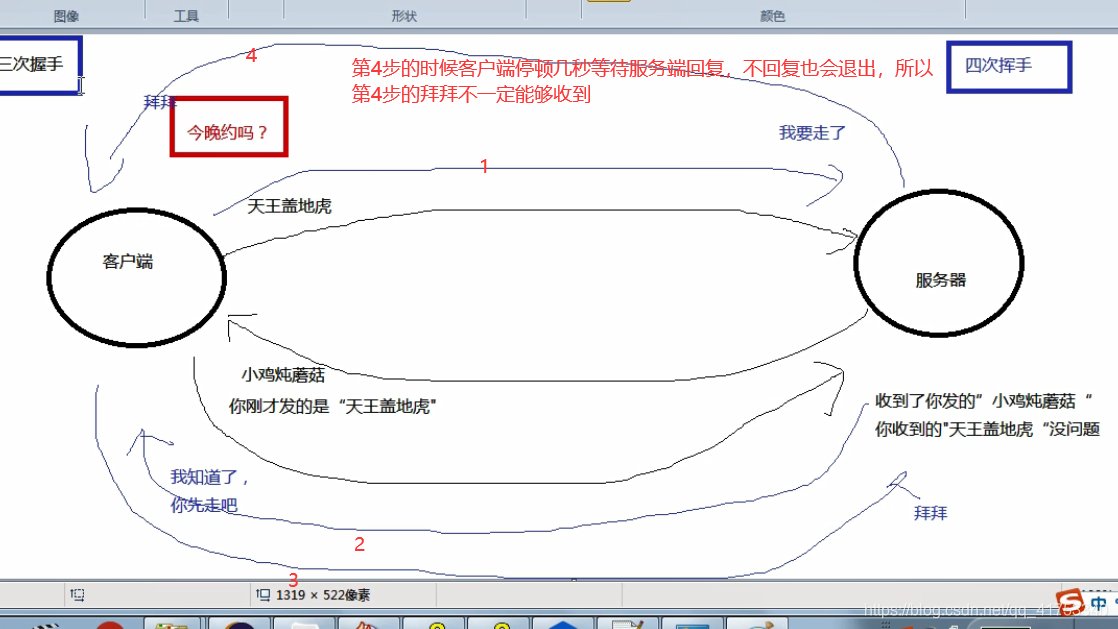
开始之前有三次握手,离开之前有四次挥手,保证传输的稳定性
注意
IP地址、端口号、网络协议、TCP、UDP代码具体实现后面文章会讲到
转载地址:http://kqgxz.baihongyu.com/